Build Earthquake Catalog
In this final notebook, we read the matched-filter database, remove the multiple detections and write a clean earthquake catalog in a csv file.
[1]:
import os
n_CPUs = 12
os.environ["OMP_NUM_THREADS"] = str(n_CPUs)
import BPMF
import glob
import h5py as h5
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import sys
from tqdm import tqdm
from time import time as give_time
[2]:
# PROGRAM PARAMETERS
NETWORK_FILENAME = "network.csv"
TEMPLATE_DB = "template_db"
MATCHED_FILTER_DB = "matched_filter_db"
CHECK_SUMMARY_FILE = False
PATH_MF = os.path.join(BPMF.cfg.OUTPUT_PATH, MATCHED_FILTER_DB)
DATA_FOLDER = "preprocessed_2_12"
[3]:
# read network metadata
net = BPMF.dataset.Network(NETWORK_FILENAME)
net.read()
Read the detected events’ metadata for each template
[4]:
# template filenames
template_filenames = glob.glob(os.path.join(BPMF.cfg.OUTPUT_PATH, TEMPLATE_DB, "template*"))
template_filenames.sort()
# initialize the template group
template_group = BPMF.dataset.TemplateGroup.read_from_files(template_filenames, net)
template_group.read_catalog(
extra_attributes=["cc"],
progress=True,
db_path=PATH_MF,
check_summary_file=CHECK_SUMMARY_FILE,
)
Reading catalog: 100%|██████████| 7/7 [00:00<00:00, 13.37it/s]
The BPMF.dataset.TemplateGroup
now has a catalog
attribute, which is a BPMF.dataset.Catalog
instance.
[5]:
template_group.catalog
[5]:
<BPMF.dataset.Catalog at 0x7f9f414557b0>
[6]:
template_group.catalog.catalog
[6]:
longitude | latitude | depth | origin_time | cc | tid | |
---|---|---|---|---|---|---|
event_id | ||||||
1.0 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 06:56:02.200 | 0.432075 | 1 |
3.0 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 06:56:02.280 | 0.289950 | 3 |
5.0 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 06:56:02.520 | 0.331015 | 5 |
2.0 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 06:56:02.560 | 0.404524 | 2 |
0.0 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 06:56:02.560 | 0.404524 | 0 |
... | ... | ... | ... | ... | ... | ... |
2.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 2 |
0.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 0 |
6.0 | 30.294482 | 40.628281 | -1.517578 | 2012-07-07 15:26:15.080 | 1.000000 | 6 |
3.14 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 16:50:34.840 | 0.255224 | 3 |
1.31 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 19:23:20.560 | 0.146581 | 1 |
148 rows × 6 columns
Remove the multiple detections
Remove multiple detections with the TemplateGroup.remove_multiples
method.
[7]:
# DISTANCE_CRITERION_KM: Distance, in km, between two detected events (within uncertainties) below which
# detected events are investigated for equality.
DISTANCE_CRITERION_KM = 15.0
# DT_CRITERION_SEC: Inter-event time, in seconds, between two detected events below which
# detected events are investigated for redundancy.
DT_CRITERION_SEC = 4.0
# SIMILARITY_CRITERION: Inter-template correlation coefficient below which detected events are investigated for equality.
SIMILARITY_CRITERION = 0.10
# N_CLOSEST_STATIONS: When computing the inter-template correlation coefficient, use the N_CLOSEST_STATIONS closest stations
# of a given pair of templates. This parameter is relevant for studies with large seismic networks.
N_CLOSEST_STATIONS = 10
[8]:
# we need to read the waveforms first
template_group.read_waveforms()
template_group.normalize(method="rms")
[9]:
template_group.remove_multiples(
n_closest_stations=N_CLOSEST_STATIONS,
dt_criterion=DT_CRITERION_SEC,
distance_criterion=DISTANCE_CRITERION_KM,
similarity_criterion=SIMILARITY_CRITERION,
progress=True,
)
/home/ebeauce/miniconda3/envs/hy7_py310/lib/python3.10/site-packages/BPMF/dataset.py:3452: RuntimeWarning: invalid value encountered in divide
unit_direction /= np.sqrt(np.sum(unit_direction**2, axis=1))[
Computing the similarity matrix...
Computing the inter-template directional errors...
Searching for events detected by multiple templates
All events occurring within 4.0 sec, with uncertainty ellipsoids closer than 15.0 km will and inter-template CC larger than 0.10 be considered the same
Removing multiples: 100%|██████████| 148/148 [00:00<00:00, 2651.52it/s]
0.06s to flag the multiples
The catalog now has three new columns: origin_time_sec
(a timestamp of origin_time
in seconds), interevent_time_sec
(template-wise computation), unique_event
.
[10]:
template_group.catalog.catalog
[10]:
longitude | latitude | depth | origin_time | cc | tid | origin_time_sec | interevent_time_sec | unique_event | |
---|---|---|---|---|---|---|---|---|---|
event_id | |||||||||
1.0 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 06:56:02.200 | 0.432075 | 1 | 1.341644e+09 | 0.00 | True |
3.0 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 06:56:02.280 | 0.289950 | 3 | 1.341644e+09 | 0.08 | False |
5.0 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 06:56:02.520 | 0.331015 | 5 | 1.341644e+09 | 0.24 | False |
2.0 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 06:56:02.560 | 0.404524 | 2 | 1.341644e+09 | 0.04 | False |
0.0 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 06:56:02.560 | 0.404524 | 0 | 1.341644e+09 | 0.00 | False |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 2 | 1.341663e+09 | 245.44 | True |
0.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 0 | 1.341663e+09 | 0.00 | False |
6.0 | 30.294482 | 40.628281 | -1.517578 | 2012-07-07 15:26:15.080 | 1.000000 | 6 | 1.341675e+09 | 11309.96 | True |
3.14 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 16:50:34.840 | 0.255224 | 3 | 1.341680e+09 | 5059.76 | True |
1.31 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 19:23:20.560 | 0.146581 | 1 | 1.341689e+09 | 9165.72 | True |
148 rows × 9 columns
The final catalog is made of the unique events only.
[11]:
template_group.catalog.catalog = template_group.catalog.catalog[template_group.catalog.catalog["unique_event"]]
Let’s add the location uncertainties from the template events.
[12]:
for tp in template_group.templates:
tid = tp.tid
selection = template_group.catalog.catalog["tid"] == tid
template_group.catalog.catalog.loc[selection, "hmax_unc"] = tp.hmax_unc
template_group.catalog.catalog.loc[selection, "hmin_unc"] = tp.hmin_unc
template_group.catalog.catalog.loc[selection, "az_hmax_unc"] = tp.az_hmax_unc
template_group.catalog.catalog.loc[selection, "vmax_unc"] = tp.vmax_unc
/tmp/ipykernel_160176/1634894027.py:4: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
template_group.catalog.catalog.loc[selection, "hmax_unc"] = tp.hmax_unc
/tmp/ipykernel_160176/1634894027.py:5: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
template_group.catalog.catalog.loc[selection, "hmin_unc"] = tp.hmin_unc
/tmp/ipykernel_160176/1634894027.py:6: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
template_group.catalog.catalog.loc[selection, "az_hmax_unc"] = tp.az_hmax_unc
/tmp/ipykernel_160176/1634894027.py:7: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
template_group.catalog.catalog.loc[selection, "vmax_unc"] = tp.vmax_unc
[13]:
print(f"There are {len(template_group.catalog.catalog)} events in our template matching catalog!")
template_group.catalog.catalog
There are 52 events in our template matching catalog!
[13]:
longitude | latitude | depth | origin_time | cc | tid | origin_time_sec | interevent_time_sec | unique_event | hmax_unc | hmin_unc | az_hmax_unc | vmax_unc | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
event_id | |||||||||||||
1.0 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 06:56:02.200 | 0.432075 | 1 | 1.341644e+09 | 0.00 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.1 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 06:56:52.160 | 0.246272 | 3 | 1.341644e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.2 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:07:45.440 | 0.403376 | 1 | 1.341645e+09 | 652.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.3 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:09:59.680 | 0.239296 | 3 | 1.341645e+09 | 133.84 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
0.3 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:10:12.440 | 0.257793 | 0 | 1.341645e+09 | 0.36 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
5.3 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:10:20.000 | 0.402187 | 5 | 1.341645e+09 | 7.56 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
5.4 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:10:39.160 | 0.377112 | 5 | 1.341645e+09 | 19.12 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.4 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:10:53.640 | 0.394400 | 1 | 1.341645e+09 | 13.60 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.4 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:11:07.280 | 0.681143 | 3 | 1.341645e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
5.6 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:11:36.080 | 0.181182 | 5 | 1.341645e+09 | 28.52 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
0.8 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:12:06.200 | 1.000000 | 0 | 1.341645e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.7 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:14:25.000 | 1.000000 | 1 | 1.341645e+09 | 138.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
1.8 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:15:44.880 | 0.183907 | 1 | 1.341645e+09 | 79.52 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.9 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:18:17.040 | 0.398268 | 5 | 1.341645e+09 | 152.16 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
0.11 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:22:16.240 | 0.178471 | 0 | 1.341646e+09 | 239.16 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
0.12 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:22:43.880 | 0.169656 | 0 | 1.341646e+09 | 27.64 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
3.6 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:23:08.320 | 0.491056 | 3 | 1.341646e+09 | 24.44 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.9 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:24:09.560 | 0.188800 | 1 | 1.341646e+09 | 60.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.11 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:24:34.000 | 1.000000 | 5 | 1.341646e+09 | 0.24 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.11 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:26:09.920 | 0.211587 | 1 | 1.341646e+09 | 95.88 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
1.12 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:27:21.560 | 0.244670 | 1 | 1.341646e+09 | 71.64 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.13 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:29:48.920 | 0.210121 | 5 | 1.341646e+09 | 147.00 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
3.8 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:34:42.600 | 0.424897 | 3 | 1.341646e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
3.9 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:35:58.000 | 0.260982 | 3 | 1.341647e+09 | 75.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
3.10 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:39:17.400 | 0.202312 | 3 | 1.341647e+09 | 199.16 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
3.11 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 08:00:23.360 | 0.199350 | 3 | 1.341648e+09 | 1265.96 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.14 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:10:46.560 | 0.158986 | 1 | 1.341649e+09 | 623.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
0.17 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:15:48.880 | 0.246277 | 0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
0.18 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:17:35.000 | 0.563850 | 0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.17 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:30:27.240 | 0.218191 | 1 | 1.341650e+09 | 772.24 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.19 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:41:19.560 | 0.304813 | 2 | 1.341650e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.19 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:45:27.600 | 0.225189 | 1 | 1.341651e+09 | 248.04 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.21 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:46:34.360 | 0.321005 | 2 | 1.341651e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.21 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:47:00.560 | 0.407170 | 1 | 1.341651e+09 | 26.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.12 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 08:48:43.800 | 1.000000 | 3 | 1.341651e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
5.21 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:00.600 | 0.390236 | 5 | 1.341652e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
5.22 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:55.640 | 0.152766 | 5 | 1.341652e+09 | 54.96 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.24 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 09:20:12.080 | 0.693933 | 1 | 1.341653e+09 | 556.44 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.24 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:27:10.120 | 0.332328 | 5 | 1.341653e+09 | 417.68 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.25 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 09:42:13.440 | 0.206876 | 1 | 1.341654e+09 | 903.28 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.27 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 09:46:04.920 | 0.341435 | 2 | 1.341654e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
5.26 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:59:27.240 | 0.186767 | 5 | 1.341655e+09 | 802.32 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.27 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 10:05:20.680 | 0.159305 | 1 | 1.341656e+09 | 353.36 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
4.0 | 30.316699 | 40.754688 | 0.285156 | 2012-07-07 10:16:39.800 | 1.000000 | 4 | 1.341656e+09 | 679.12 | True | 3.378762 | 1.691182 | -143.772340 | 2.733743 |
5.27 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 10:41:34.400 | 0.335804 | 5 | 1.341658e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
0.30 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:15:32.640 | 0.298780 | 0 | 1.341660e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
0.31 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:23:41.520 | 0.385650 | 0 | 1.341660e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.30 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 12:13:39.320 | 0.266582 | 1 | 1.341663e+09 | 2997.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 2 | 1.341663e+09 | 245.44 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
6.0 | 30.294482 | 40.628281 | -1.517578 | 2012-07-07 15:26:15.080 | 1.000000 | 6 | 1.341675e+09 | 11309.96 | True | 4.898208 | 2.005268 | 177.708818 | 3.423410 |
3.14 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 16:50:34.840 | 0.255224 | 3 | 1.341680e+09 | 5059.76 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.31 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 19:23:20.560 | 0.146581 | 1 | 1.341689e+09 | 9165.72 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
Let’s plot these events on a map. You will see that there are far fewer dots on the map than the total number of earthquakes in our catalog… This is because all newly detected events are attributed their template locations. Therefore, most events are plotted at the exact same location.
[14]:
fig = template_group.catalog.plot_map(
figsize=(10, 10), network=net, s=50, markersize_station=50, lat_margin=0.02, plot_uncertainties=False
)
ax = fig.get_axes()[0]
ax.set_facecolor("dimgrey")
ax.patch.set_alpha(0.15)
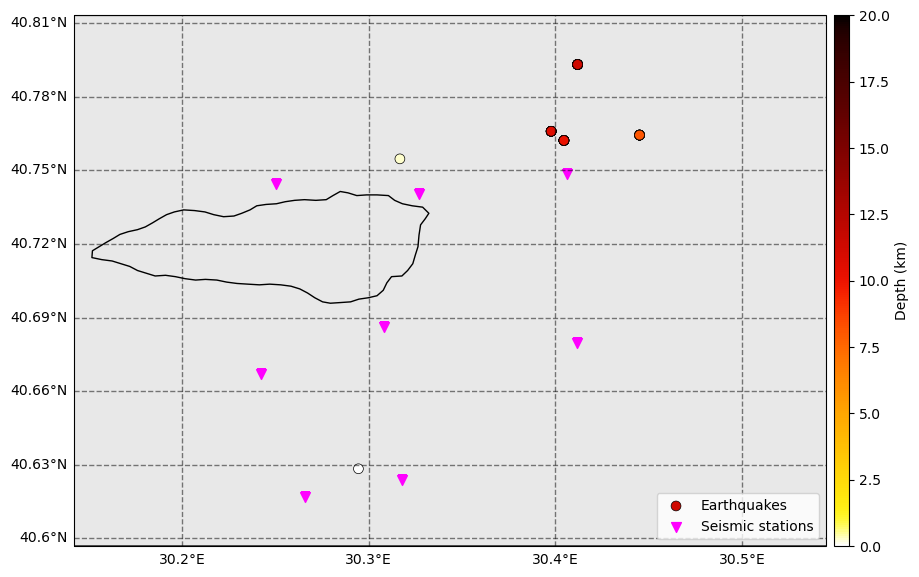
Bonus: Relocate each events
In your workflow, you might be ok with the approximate locations of the template matching catalog. However, if you decide to keep refining the catalog, here are some suggestions to get started.
One possibility to start refining the locations of all events is to re-run the same process as in notebook 6, namely PhaseNet
and NonLinLoc
.
[15]:
import tensorflow as tf
from BPMF.data_reader_examples import data_reader_mseed
# this is necessary to limit the number of threads spawn by tf
os.environ["TF_NUM_INTRAOP_THREADS"] = str(n_CPUs)
os.environ["TF_NUM_INTEROP_THREADS"] = str(n_CPUs)
tf.config.threading.set_inter_op_parallelism_threads(n_CPUs)
tf.config.threading.set_intra_op_parallelism_threads(n_CPUs)
tf.config.set_soft_device_placement(True)
2023-03-08 16:11:21.120889: W tensorflow/stream_executor/platform/default/dso_loader.cc:64] Could not load dynamic library 'libcudart.so.11.0'; dlerror: libcudart.so.11.0: cannot open shared object file: No such file or directory; LD_LIBRARY_PATH: /usr/local/cuda-11.7/lib64
2023-03-08 16:11:21.120935: I tensorflow/stream_executor/cuda/cudart_stub.cc:29] Ignore above cudart dlerror if you do not have a GPU set up on your machine.
[16]:
# PhaseNet picking parameters
# PhaseNet was trained for 100Hz data. Even if we saw that running PhaseNet on 25Hz data
# was good for backprojection, here, picking benefits from running PhaseNet on 100Hz data.
# Thus, we will upsample the waveforms before running PhaseNet.
PHASENET_SAMPLING_RATE_HZ = 100.
UPSAMPLING_BEFORE_PN_RELOCATION = int(PHASENET_SAMPLING_RATE_HZ/BPMF.cfg.SAMPLING_RATE_HZ)
DOWNSAMPLING_BEFORE_PN_RELOCATION = 1
# DURATION_SEC: the duration, in seconds, of the data stream starting at the detection time
# defined by Event.origin_time. This data stream is used for picking the P/S waves.
DURATION_SEC = 60.0
# THRESHOLD_P: probability of P-wave arrival above which we declare a pick. If several picks are
# declared during the DURATION_SEC data stream, we only keep the best one. We can
# afford using a low probability threshold since we already know with some confidence
# that an earthquake is in the data stream.
THRESHOLD_P = 0.10
# THRESHOLD_S: probability of S-wave arrival above which we declare a pick.
THRESHOLD_S = 0.10
# DATA_FOLDER: name of the folder where the waveforms we want to use for picking are stored
DATA_FOLDER = "preprocessed_2_12"
# COMPONENT_ALIASES: A dictionary that defines the possible channel names to search for
# for example, the seismometer might not be oriented and the horizontal channels
# might be named 1 and 2, in which case we arbitrarily decide to take 1 as the "N" channel
# and 2 as the "E" channel. This doesn't matter for picking P- and S-wave arrival times.
COMPONENT_ALIASES = {"N": ["N", "1"], "E": ["E", "2"], "Z": ["Z"]}
# PHASE_ON_COMP: dictionary defining which moveout we use to extract the waveform
PHASE_ON_COMP = {"N": "S", "1": "S", "E": "S", "2": "S", "Z": "P"}
# USE_APRIORI_PICKS: boolean. This option is IMPORTANT when running BPMF in HIGH SEISMICITY CONTEXTS, like
# during the aftershock sequence of a large earthquake. If there are many events happening
# close to each other in time, we need to guide PhaseNet to pick the right set of picks.
# For that, we use the predicted P- and S-wave times from backprojection to add extra weight to
# the picks closer to those times and make it more likely to identify them as the "best" picks.
# WARNING: If there are truly many events, even this trick might fail. It's because "phase association"
# is an intrinsically hard problem in this case, and the picking might be hard to do automatically.
USE_APRIORI_PICKS = True
# MAX_HORIZONTAL_UNC_KM: Horizontal location uncertainty, in km, above which we keep the template location
MAX_HORIZONTAL_UNC_KM = 10.
# location parameters
LOCATION_ROUTINE = "NLLoc"
# NLLOC_METHOD: string that defines what loss function is used by NLLoc, see http://alomax.free.fr/nlloc/ for more info.
# Using some flavor of 'EDT' is important to obtain robust locations that are not sensitive to pick outliers.
NLLOC_METHOD = "EDT"
# MINIMUM_NUM_STATIONS_W_PICKS: minimum number of stations with picks to even try relocation.
MINIMUM_NUM_STATIONS_W_PICKS = 3
# we set a maximum tolerable difference, in percentage, between the picked time and the predicted travel time
MAX_TIME_DIFFERENT_PICKS_PREDICTED_PERCENT = 10
[17]:
events = {}
for idx, row in template_group.catalog.catalog.iterrows():
tid, evidx = row.name.split(".")
# get the template instance from template_group
template = template_group.templates[template_group.tindexes.loc[int(tid)]]
# this is the filename of the database where template tid's detected events were stored
detection_db_filename = f"detections_template{tid}.h5"
db_path = os.path.join(BPMF.cfg.OUTPUT_PATH, MATCHED_FILTER_DB)
with h5.File(os.path.join(db_path, detection_db_filename), mode="r") as fdet:
keys = list(fdet.keys())
event = BPMF.dataset.Event.read_from_file(
hdf5_file=fdet[keys[int(evidx)]], data_reader=data_reader_mseed
)
# # attach data reader this way (note: conflict with data_reader argument in phasenet's wrapper module)
# event.data_reader = data_reader_mseed
# pick P-/S-wave arrivals
event.pick_PS_phases(
DURATION_SEC,
phase_on_comp=PHASE_ON_COMP,
threshold_P=THRESHOLD_P,
threshold_S=THRESHOLD_S,
component_aliases=COMPONENT_ALIASES,
inter_op_parallelism_threads=n_CPUs,
intra_op_parallelism_threads=n_CPUs,
data_folder=DATA_FOLDER,
upsampling=UPSAMPLING_BEFORE_PN_RELOCATION,
downsampling=DOWNSAMPLING_BEFORE_PN_RELOCATION,
use_apriori_picks=USE_APRIORI_PICKS,
)
if len(event.picks.dropna(how="all")) >= MINIMUM_NUM_STATIONS_W_PICKS:
# first relocation, insensitive to outliers
event.relocate(
stations=net.stations, routine=LOCATION_ROUTINE, method=NLLOC_METHOD,
)
if "NLLoc_reloc" in event.aux_data:
# this variable was inserted into ev.aux_data if NLLoc successfully located the event
# use predicted times to remove outlier picks
event.remove_outlier_picks(max_diff_percent=MAX_TIME_DIFFERENT_PICKS_PREDICTED_PERCENT)
if len(event.picks.dropna(how="all")) >= MINIMUM_NUM_STATIONS_W_PICKS:
# first relocation, insensitive to outliers
event.relocate(
stations=net.stations, routine=LOCATION_ROUTINE, method=NLLOC_METHOD,
)
else:
del event.aux_data["NLLoc_reloc"]
events[row.name] = event
if ("NLLoc_reloc" in event.aux_data) and (event.hmax_unc) < MAX_HORIZONTAL_UNC_KM:
template_group.catalog.catalog.loc[row.name, "longitude"] = event.longitude
template_group.catalog.catalog.loc[row.name, "latitude"] = event.latitude
template_group.catalog.catalog.loc[row.name, "depth"] = event.depth
n events: 1, n stations: 8, batch size (n events x n stations): 8
2023-03-08 16:11:25.822008: W tensorflow/stream_executor/platform/default/dso_loader.cc:64] Could not load dynamic library 'libcuda.so.1'; dlerror: libcuda.so.1: cannot open shared object file: No such file or directory; LD_LIBRARY_PATH: /usr/local/cuda-11.7/lib64
2023-03-08 16:11:25.822047: W tensorflow/stream_executor/cuda/cuda_driver.cc:269] failed call to cuInit: UNKNOWN ERROR (303)
2023-03-08 16:11:25.822072: I tensorflow/stream_executor/cuda/cuda_diagnostics.cc:156] kernel driver does not appear to be running on this host (hypo-7): /proc/driver/nvidia/version does not exist
2023-03-08 16:11:25.822308: I tensorflow/core/platform/cpu_feature_guard.cc:193] This TensorFlow binary is optimized with oneAPI Deep Neural Network Library (oneDNN) to use the following CPU instructions in performance-critical operations: AVX2 FMA
To enable them in other operations, rebuild TensorFlow with the appropriate compiler flags.
2023-03-08 16:11:26.534948: I tensorflow/compiler/mlir/mlir_graph_optimization_pass.cc:354] MLIR V1 optimization pass is not enabled
Pred: 100%|██████████| 1/1 [00:00<00:00, 4.32it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.37it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 4.79it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.25it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.29it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.56it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.36it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.44it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.89it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 3.87it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.72it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.49it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.48it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.35it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.24it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.31it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.30it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.47it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.53it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.51it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.18it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.46it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.31it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.03it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.19it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.18it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.65it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 4.21it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.29it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.33it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.33it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.34it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.27it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.57it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.45it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.48it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.38it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.21it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 3.96it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.46it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.37it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.31it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.54it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.54it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.74it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.55it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.11it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.36it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.44it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.15it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.65it/s]
n events: 1, n stations: 8, batch size (n events x n stations): 8
Pred: 100%|██████████| 1/1 [00:00<00:00, 5.49it/s]
[18]:
fig = template_group.catalog.plot_map(
figsize=(10, 10), network=net, s=50, markersize_station=50, lat_margin=0.02, plot_uncertainties=False
)
ax = fig.get_axes()[0]
ax.set_facecolor("dimgrey")
ax.patch.set_alpha(0.15)
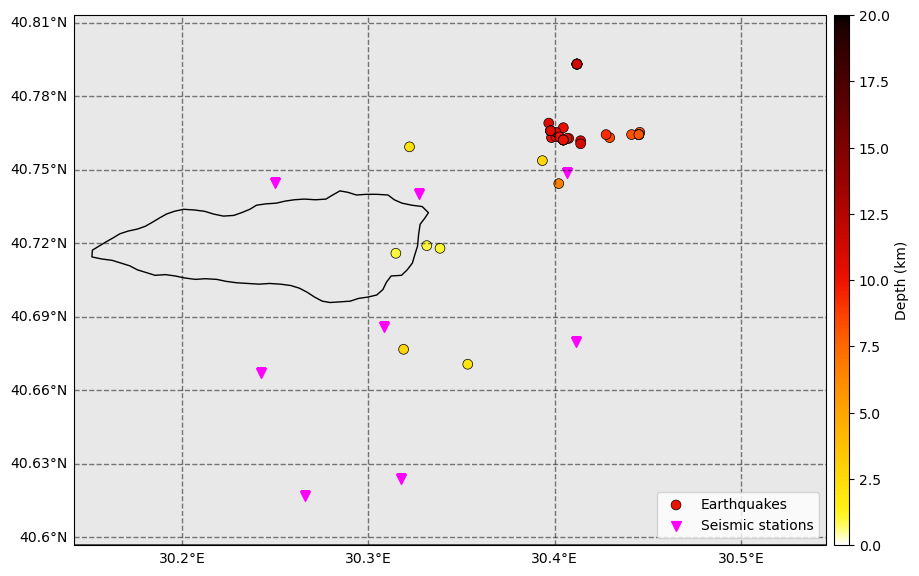
[19]:
template_group.catalog.catalog
[19]:
longitude | latitude | depth | origin_time | cc | tid | origin_time_sec | interevent_time_sec | unique_event | hmax_unc | hmin_unc | az_hmax_unc | vmax_unc | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
event_id | |||||||||||||
1.0 | 30.399707 | 40.764062 | 10.136719 | 2012-07-07 06:56:02.200 | 0.432075 | 1 | 1.341644e+09 | 0.00 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.1 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 06:56:52.160 | 0.246272 | 3 | 1.341644e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.2 | 30.404590 | 40.767187 | 10.238281 | 2012-07-07 07:07:45.440 | 0.403376 | 1 | 1.341645e+09 | 652.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.3 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:09:59.680 | 0.239296 | 3 | 1.341645e+09 | 133.84 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
0.3 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:10:12.440 | 0.257793 | 0 | 1.341645e+09 | 0.36 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
5.3 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:10:20.000 | 0.402187 | 5 | 1.341645e+09 | 7.56 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
5.4 | 30.398242 | 40.763125 | 10.289062 | 2012-07-07 07:10:39.160 | 0.377112 | 5 | 1.341645e+09 | 19.12 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.4 | 30.413867 | 40.761875 | 10.898438 | 2012-07-07 07:10:53.640 | 0.394400 | 1 | 1.341645e+09 | 13.60 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.4 | 30.413867 | 40.760625 | 10.898438 | 2012-07-07 07:11:07.280 | 0.681143 | 3 | 1.341645e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
5.6 | 30.407520 | 40.762812 | 10.136719 | 2012-07-07 07:11:36.080 | 0.181182 | 5 | 1.341645e+09 | 28.52 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
0.8 | 30.406543 | 40.762812 | 10.238281 | 2012-07-07 07:12:06.200 | 1.000000 | 0 | 1.341645e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.7 | 30.400684 | 40.763437 | 10.136719 | 2012-07-07 07:14:25.000 | 1.000000 | 1 | 1.341645e+09 | 138.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
1.8 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:15:44.880 | 0.183907 | 1 | 1.341645e+09 | 79.52 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.9 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:18:17.040 | 0.398268 | 5 | 1.341645e+09 | 152.16 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
0.11 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:22:16.240 | 0.178471 | 0 | 1.341646e+09 | 239.16 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
0.12 | 30.402148 | 40.744375 | 6.632812 | 2012-07-07 07:22:43.880 | 0.169656 | 0 | 1.341646e+09 | 27.64 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
3.6 | 30.393359 | 40.753750 | 2.671875 | 2012-07-07 07:23:08.320 | 0.491056 | 3 | 1.341646e+09 | 24.44 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.9 | 30.396777 | 40.769062 | 10.746094 | 2012-07-07 07:24:09.560 | 0.188800 | 1 | 1.341646e+09 | 60.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.11 | 30.400684 | 40.765312 | 10.746094 | 2012-07-07 07:24:34.000 | 1.000000 | 5 | 1.341646e+09 | 0.24 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.11 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:26:09.920 | 0.211587 | 1 | 1.341646e+09 | 95.88 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
1.12 | 30.314746 | 40.715938 | 0.996094 | 2012-07-07 07:27:21.560 | 0.244670 | 1 | 1.341646e+09 | 71.64 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.13 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:29:48.920 | 0.210121 | 5 | 1.341646e+09 | 147.00 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
3.8 | 30.445605 | 40.765312 | 8.003906 | 2012-07-07 07:34:42.600 | 0.424897 | 3 | 1.341646e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
3.9 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:35:58.000 | 0.260982 | 3 | 1.341647e+09 | 75.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
3.10 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:39:17.400 | 0.202312 | 3 | 1.341647e+09 | 199.16 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
3.11 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 08:00:23.360 | 0.199350 | 3 | 1.341648e+09 | 1265.96 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.14 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:10:46.560 | 0.158986 | 1 | 1.341649e+09 | 623.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
0.17 | 30.338428 | 40.717969 | 1.021484 | 2012-07-07 08:15:48.880 | 0.246277 | 0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
0.18 | 30.429492 | 40.763125 | 8.460938 | 2012-07-07 08:17:35.000 | 0.563850 | 0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.17 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:30:27.240 | 0.218191 | 1 | 1.341650e+09 | 772.24 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.19 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:41:19.560 | 0.304813 | 2 | 1.341650e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.19 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:45:27.600 | 0.225189 | 1 | 1.341651e+09 | 248.04 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.21 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:46:34.360 | 0.321005 | 2 | 1.341651e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.21 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:47:00.560 | 0.407170 | 1 | 1.341651e+09 | 26.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3.12 | 30.441211 | 40.764375 | 8.257812 | 2012-07-07 08:48:43.800 | 1.000000 | 3 | 1.341651e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
5.21 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:00.600 | 0.390236 | 5 | 1.341652e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
5.22 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:55.640 | 0.152766 | 5 | 1.341652e+09 | 54.96 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.24 | 30.402637 | 40.763437 | 9.933594 | 2012-07-07 09:20:12.080 | 0.693933 | 1 | 1.341653e+09 | 556.44 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
5.24 | 30.353320 | 40.670625 | 1.960938 | 2012-07-07 09:27:10.120 | 0.332328 | 5 | 1.341653e+09 | 417.68 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.25 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 09:42:13.440 | 0.206876 | 1 | 1.341654e+09 | 903.28 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.27 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 09:46:04.920 | 0.341435 | 2 | 1.341654e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
5.26 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:59:27.240 | 0.186767 | 5 | 1.341655e+09 | 802.32 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
1.27 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 10:05:20.680 | 0.159305 | 1 | 1.341656e+09 | 353.36 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
4.0 | 30.322070 | 40.759375 | 2.164062 | 2012-07-07 10:16:39.800 | 1.000000 | 4 | 1.341656e+09 | 679.12 | True | 3.378762 | 1.691182 | -143.772340 | 2.733743 |
5.27 | 30.427539 | 40.764375 | 9.273438 | 2012-07-07 10:41:34.400 | 0.335804 | 5 | 1.341658e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
0.30 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:15:32.640 | 0.298780 | 0 | 1.341660e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
0.31 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:23:41.520 | 0.385650 | 0 | 1.341660e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
1.30 | 30.331348 | 40.719062 | 0.996094 | 2012-07-07 12:13:39.320 | 0.266582 | 1 | 1.341663e+09 | 2997.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
2.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 2 | 1.341663e+09 | 245.44 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
6.0 | 30.318896 | 40.676719 | 2.697266 | 2012-07-07 15:26:15.080 | 1.000000 | 6 | 1.341675e+09 | 11309.96 | True | 4.898208 | 2.005268 | 177.708818 | 3.423410 |
3.14 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 16:50:34.840 | 0.255224 | 3 | 1.341680e+09 | 5059.76 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
1.31 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 19:23:20.560 | 0.146581 | 1 | 1.341689e+09 | 9165.72 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
Assemble the backprojection and template matching catalog
When selecting the template events from the backprojection catalog, we imposed some quality criteria that might have thrown out some events that we still want in our final catalog. Here, we make a simple comparison of the backprojection and template matching catalogs to find these missing events and add them to the final catalog.
[20]:
BACKPROJECTION_CATALOG_FILENAME = "backprojection_catalog.csv"
[21]:
tm_catalog = template_group.catalog.catalog.copy()
tm_catalog.reset_index(inplace=True)
for i in range(len(tm_catalog)):
tm_catalog.loc[i, "event_id"] = f"tm_{tm_catalog.loc[i, 'event_id']}"
tm_catalog
[21]:
event_id | longitude | latitude | depth | origin_time | cc | tid | origin_time_sec | interevent_time_sec | unique_event | hmax_unc | hmin_unc | az_hmax_unc | vmax_unc | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | tm_1.0 | 30.399707 | 40.764062 | 10.136719 | 2012-07-07 06:56:02.200 | 0.432075 | 1 | 1.341644e+09 | 0.00 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
1 | tm_3.1 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 06:56:52.160 | 0.246272 | 3 | 1.341644e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
2 | tm_1.2 | 30.404590 | 40.767187 | 10.238281 | 2012-07-07 07:07:45.440 | 0.403376 | 1 | 1.341645e+09 | 652.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
3 | tm_3.3 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:09:59.680 | 0.239296 | 3 | 1.341645e+09 | 133.84 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
4 | tm_0.3 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:10:12.440 | 0.257793 | 0 | 1.341645e+09 | 0.36 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
5 | tm_5.3 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:10:20.000 | 0.402187 | 5 | 1.341645e+09 | 7.56 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
6 | tm_5.4 | 30.398242 | 40.763125 | 10.289062 | 2012-07-07 07:10:39.160 | 0.377112 | 5 | 1.341645e+09 | 19.12 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
7 | tm_1.4 | 30.413867 | 40.761875 | 10.898438 | 2012-07-07 07:10:53.640 | 0.394400 | 1 | 1.341645e+09 | 13.60 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
8 | tm_3.4 | 30.413867 | 40.760625 | 10.898438 | 2012-07-07 07:11:07.280 | 0.681143 | 3 | 1.341645e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
9 | tm_5.6 | 30.407520 | 40.762812 | 10.136719 | 2012-07-07 07:11:36.080 | 0.181182 | 5 | 1.341645e+09 | 28.52 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
10 | tm_0.8 | 30.406543 | 40.762812 | 10.238281 | 2012-07-07 07:12:06.200 | 1.000000 | 0 | 1.341645e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
11 | tm_1.7 | 30.400684 | 40.763437 | 10.136719 | 2012-07-07 07:14:25.000 | 1.000000 | 1 | 1.341645e+09 | 138.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
12 | tm_1.8 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:15:44.880 | 0.183907 | 1 | 1.341645e+09 | 79.52 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
13 | tm_5.9 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:18:17.040 | 0.398268 | 5 | 1.341645e+09 | 152.16 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
14 | tm_0.11 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:22:16.240 | 0.178471 | 0 | 1.341646e+09 | 239.16 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
15 | tm_0.12 | 30.402148 | 40.744375 | 6.632812 | 2012-07-07 07:22:43.880 | 0.169656 | 0 | 1.341646e+09 | 27.64 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
16 | tm_3.6 | 30.393359 | 40.753750 | 2.671875 | 2012-07-07 07:23:08.320 | 0.491056 | 3 | 1.341646e+09 | 24.44 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
17 | tm_1.9 | 30.396777 | 40.769062 | 10.746094 | 2012-07-07 07:24:09.560 | 0.188800 | 1 | 1.341646e+09 | 60.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
18 | tm_5.11 | 30.400684 | 40.765312 | 10.746094 | 2012-07-07 07:24:34.000 | 1.000000 | 5 | 1.341646e+09 | 0.24 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
19 | tm_1.11 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:26:09.920 | 0.211587 | 1 | 1.341646e+09 | 95.88 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
20 | tm_1.12 | 30.314746 | 40.715938 | 0.996094 | 2012-07-07 07:27:21.560 | 0.244670 | 1 | 1.341646e+09 | 71.64 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
21 | tm_5.13 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:29:48.920 | 0.210121 | 5 | 1.341646e+09 | 147.00 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
22 | tm_3.8 | 30.445605 | 40.765312 | 8.003906 | 2012-07-07 07:34:42.600 | 0.424897 | 3 | 1.341646e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
23 | tm_3.9 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:35:58.000 | 0.260982 | 3 | 1.341647e+09 | 75.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
24 | tm_3.10 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:39:17.400 | 0.202312 | 3 | 1.341647e+09 | 199.16 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
25 | tm_3.11 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 08:00:23.360 | 0.199350 | 3 | 1.341648e+09 | 1265.96 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
26 | tm_1.14 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:10:46.560 | 0.158986 | 1 | 1.341649e+09 | 623.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
27 | tm_0.17 | 30.338428 | 40.717969 | 1.021484 | 2012-07-07 08:15:48.880 | 0.246277 | 0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
28 | tm_0.18 | 30.429492 | 40.763125 | 8.460938 | 2012-07-07 08:17:35.000 | 0.563850 | 0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
29 | tm_1.17 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:30:27.240 | 0.218191 | 1 | 1.341650e+09 | 772.24 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
30 | tm_2.19 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:41:19.560 | 0.304813 | 2 | 1.341650e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
31 | tm_1.19 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:45:27.600 | 0.225189 | 1 | 1.341651e+09 | 248.04 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
32 | tm_2.21 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:46:34.360 | 0.321005 | 2 | 1.341651e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
33 | tm_1.21 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:47:00.560 | 0.407170 | 1 | 1.341651e+09 | 26.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
34 | tm_3.12 | 30.441211 | 40.764375 | 8.257812 | 2012-07-07 08:48:43.800 | 1.000000 | 3 | 1.341651e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
35 | tm_5.21 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:00.600 | 0.390236 | 5 | 1.341652e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
36 | tm_5.22 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:55.640 | 0.152766 | 5 | 1.341652e+09 | 54.96 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
37 | tm_1.24 | 30.402637 | 40.763437 | 9.933594 | 2012-07-07 09:20:12.080 | 0.693933 | 1 | 1.341653e+09 | 556.44 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
38 | tm_5.24 | 30.353320 | 40.670625 | 1.960938 | 2012-07-07 09:27:10.120 | 0.332328 | 5 | 1.341653e+09 | 417.68 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
39 | tm_1.25 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 09:42:13.440 | 0.206876 | 1 | 1.341654e+09 | 903.28 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
40 | tm_2.27 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 09:46:04.920 | 0.341435 | 2 | 1.341654e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
41 | tm_5.26 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:59:27.240 | 0.186767 | 5 | 1.341655e+09 | 802.32 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
42 | tm_1.27 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 10:05:20.680 | 0.159305 | 1 | 1.341656e+09 | 353.36 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
43 | tm_4.0 | 30.322070 | 40.759375 | 2.164062 | 2012-07-07 10:16:39.800 | 1.000000 | 4 | 1.341656e+09 | 679.12 | True | 3.378762 | 1.691182 | -143.772340 | 2.733743 |
44 | tm_5.27 | 30.427539 | 40.764375 | 9.273438 | 2012-07-07 10:41:34.400 | 0.335804 | 5 | 1.341658e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 |
45 | tm_0.30 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:15:32.640 | 0.298780 | 0 | 1.341660e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
46 | tm_0.31 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:23:41.520 | 0.385650 | 0 | 1.341660e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
47 | tm_1.30 | 30.331348 | 40.719062 | 0.996094 | 2012-07-07 12:13:39.320 | 0.266582 | 1 | 1.341663e+09 | 2997.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
48 | tm_2.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 2 | 1.341663e+09 | 245.44 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 |
49 | tm_6.0 | 30.318896 | 40.676719 | 2.697266 | 2012-07-07 15:26:15.080 | 1.000000 | 6 | 1.341675e+09 | 11309.96 | True | 4.898208 | 2.005268 | 177.708818 | 3.423410 |
50 | tm_3.14 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 16:50:34.840 | 0.255224 | 3 | 1.341680e+09 | 5059.76 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 |
51 | tm_1.31 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 19:23:20.560 | 0.146581 | 1 | 1.341689e+09 | 9165.72 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 |
[22]:
bp_catalog = pd.read_csv(
os.path.join(BPMF.cfg.OUTPUT_PATH, BACKPROJECTION_CATALOG_FILENAME),
index_col=0
)
# add event ids
for i in range(len(bp_catalog)):
bp_catalog.loc[i, "event_id"] = f"bp_{i}"
# convert origin times from string to pandas.Timestamp
bp_catalog["origin_time"] = pd.to_datetime(bp_catalog["origin_time"])
bp_catalog
[22]:
longitude | latitude | depth | origin_time | hmax_unc | hmin_unc | az_hmax_unc | Mw | Mw_err | event_id | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 30.402637 | 40.765937 | 10.238281 | 2012-07-07 06:56:02.560 | 1.554406 | 1.320863 | -167.389837 | 2.688609 | 2.603238e-02 | bp_0 |
1 | 30.408496 | 40.765937 | 10.238281 | 2012-07-07 07:07:45.720 | 1.386711 | 1.049999 | 148.121750 | 3.912620 | 1.835205e-31 | bp_1 |
2 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:12:06.200 | 1.204078 | 0.954942 | 138.520075 | NaN | NaN | bp_2 |
3 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:14:25.000 | 1.886675 | 1.435720 | -163.098388 | 2.809426 | 1.748471e-02 | bp_3 |
4 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:24:34.000 | 1.477016 | 1.028668 | 137.014524 | 2.604610 | 2.720755e-02 | bp_4 |
5 | 30.409961 | 40.768125 | 10.289062 | 2012-07-07 07:34:42.800 | 2.157981 | 1.659802 | 125.916805 | NaN | NaN | bp_5 |
6 | 30.413867 | 40.759375 | 9.273438 | 2012-07-07 08:17:35.080 | 1.807823 | 1.109721 | 70.558859 | NaN | NaN | bp_6 |
7 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 08:48:43.800 | 2.982386 | 1.933982 | -169.556250 | NaN | NaN | bp_7 |
8 | 30.402637 | 40.764687 | 10.035156 | 2012-07-07 09:20:12.480 | 1.116928 | 0.884104 | 159.472964 | NaN | NaN | bp_8 |
9 | 30.316699 | 40.754688 | 0.285156 | 2012-07-07 10:16:39.800 | 3.378762 | 1.691182 | -143.772340 | NaN | NaN | bp_9 |
10 | 30.421680 | 40.764375 | 9.273438 | 2012-07-07 10:41:34.320 | 1.921096 | 1.396241 | 81.876681 | NaN | NaN | bp_10 |
11 | 30.294482 | 40.628281 | -1.517578 | 2012-07-07 15:26:15.080 | 4.898208 | 2.005268 | 177.708818 | NaN | NaN | bp_11 |
[23]:
# catalog merging parameters
dt_criterion_pd = pd.Timedelta(DT_CRITERION_SEC, "s")
HMAX_UNC_CRITERION_KM = 10.
[24]:
final_catalog = tm_catalog.copy()
missing_event = np.zeros(len(bp_catalog), dtype=bool)
for i in tqdm(range(len(bp_catalog)), desc="Loop through BP cat"):
if bp_catalog.iloc[i]["hmax_unc"] > HMAX_UNC_CRITERION_KM:
continue
t_min = bp_catalog.iloc[i]["origin_time"] - dt_criterion_pd
t_max = bp_catalog.iloc[i]["origin_time"] + dt_criterion_pd
subset_tm = (tm_catalog["origin_time"] > t_min) & (tm_catalog["origin_time"] < t_max)
if np.sum(subset_tm) == 0:
missing_event[i] = True
final_catalog = pd.concat(
(tm_catalog, bp_catalog[missing_event]),
ignore_index=True
)
final_catalog.sort_values(
"origin_time", ascending=True, inplace=True
)
Loop through BP cat: 100%|██████████| 12/12 [00:00<00:00, 1574.19it/s]
[25]:
final_catalog.set_index("event_id", drop=False, inplace=True)
final_catalog
[25]:
event_id | longitude | latitude | depth | origin_time | cc | tid | origin_time_sec | interevent_time_sec | unique_event | hmax_unc | hmin_unc | az_hmax_unc | vmax_unc | Mw | Mw_err | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
event_id | ||||||||||||||||
tm_1.0 | tm_1.0 | 30.399707 | 40.764062 | 10.136719 | 2012-07-07 06:56:02.200 | 0.432075 | 1.0 | 1.341644e+09 | 0.00 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_3.1 | tm_3.1 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 06:56:52.160 | 0.246272 | 3.0 | 1.341644e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_1.2 | tm_1.2 | 30.404590 | 40.767187 | 10.238281 | 2012-07-07 07:07:45.440 | 0.403376 | 1.0 | 1.341645e+09 | 652.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_3.3 | tm_3.3 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:09:59.680 | 0.239296 | 3.0 | 1.341645e+09 | 133.84 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_0.3 | tm_0.3 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:10:12.440 | 0.257793 | 0.0 | 1.341645e+09 | 0.36 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_5.3 | tm_5.3 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:10:20.000 | 0.402187 | 5.0 | 1.341645e+09 | 7.56 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_5.4 | tm_5.4 | 30.398242 | 40.763125 | 10.289062 | 2012-07-07 07:10:39.160 | 0.377112 | 5.0 | 1.341645e+09 | 19.12 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_1.4 | tm_1.4 | 30.413867 | 40.761875 | 10.898438 | 2012-07-07 07:10:53.640 | 0.394400 | 1.0 | 1.341645e+09 | 13.60 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_3.4 | tm_3.4 | 30.413867 | 40.760625 | 10.898438 | 2012-07-07 07:11:07.280 | 0.681143 | 3.0 | 1.341645e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_5.6 | tm_5.6 | 30.407520 | 40.762812 | 10.136719 | 2012-07-07 07:11:36.080 | 0.181182 | 5.0 | 1.341645e+09 | 28.52 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_0.8 | tm_0.8 | 30.406543 | 40.762812 | 10.238281 | 2012-07-07 07:12:06.200 | 1.000000 | 0.0 | 1.341645e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_1.7 | tm_1.7 | 30.400684 | 40.763437 | 10.136719 | 2012-07-07 07:14:25.000 | 1.000000 | 1.0 | 1.341645e+09 | 138.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_1.8 | tm_1.8 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:15:44.880 | 0.183907 | 1.0 | 1.341645e+09 | 79.52 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_5.9 | tm_5.9 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:18:17.040 | 0.398268 | 5.0 | 1.341645e+09 | 152.16 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_0.11 | tm_0.11 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 07:22:16.240 | 0.178471 | 0.0 | 1.341646e+09 | 239.16 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_0.12 | tm_0.12 | 30.402148 | 40.744375 | 6.632812 | 2012-07-07 07:22:43.880 | 0.169656 | 0.0 | 1.341646e+09 | 27.64 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_3.6 | tm_3.6 | 30.393359 | 40.753750 | 2.671875 | 2012-07-07 07:23:08.320 | 0.491056 | 3.0 | 1.341646e+09 | 24.44 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_1.9 | tm_1.9 | 30.396777 | 40.769062 | 10.746094 | 2012-07-07 07:24:09.560 | 0.188800 | 1.0 | 1.341646e+09 | 60.96 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_5.11 | tm_5.11 | 30.400684 | 40.765312 | 10.746094 | 2012-07-07 07:24:34.000 | 1.000000 | 5.0 | 1.341646e+09 | 0.24 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_1.11 | tm_1.11 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 07:26:09.920 | 0.211587 | 1.0 | 1.341646e+09 | 95.88 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_1.12 | tm_1.12 | 30.314746 | 40.715938 | 0.996094 | 2012-07-07 07:27:21.560 | 0.244670 | 1.0 | 1.341646e+09 | 71.64 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_5.13 | tm_5.13 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 07:29:48.920 | 0.210121 | 5.0 | 1.341646e+09 | 147.00 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_3.8 | tm_3.8 | 30.445605 | 40.765312 | 8.003906 | 2012-07-07 07:34:42.600 | 0.424897 | 3.0 | 1.341646e+09 | 0.04 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_3.9 | tm_3.9 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:35:58.000 | 0.260982 | 3.0 | 1.341647e+09 | 75.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_3.10 | tm_3.10 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 07:39:17.400 | 0.202312 | 3.0 | 1.341647e+09 | 199.16 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_3.11 | tm_3.11 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 08:00:23.360 | 0.199350 | 3.0 | 1.341648e+09 | 1265.96 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_1.14 | tm_1.14 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:10:46.560 | 0.158986 | 1.0 | 1.341649e+09 | 623.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_0.17 | tm_0.17 | 30.338428 | 40.717969 | 1.021484 | 2012-07-07 08:15:48.880 | 0.246277 | 0.0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_0.18 | tm_0.18 | 30.429492 | 40.763125 | 8.460938 | 2012-07-07 08:17:35.000 | 0.563850 | 0.0 | 1.341649e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_1.17 | tm_1.17 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:30:27.240 | 0.218191 | 1.0 | 1.341650e+09 | 772.24 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_2.19 | tm_2.19 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:41:19.560 | 0.304813 | 2.0 | 1.341650e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_1.19 | tm_1.19 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:45:27.600 | 0.225189 | 1.0 | 1.341651e+09 | 248.04 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_2.21 | tm_2.21 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 08:46:34.360 | 0.321005 | 2.0 | 1.341651e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_1.21 | tm_1.21 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 08:47:00.560 | 0.407170 | 1.0 | 1.341651e+09 | 26.20 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_3.12 | tm_3.12 | 30.441211 | 40.764375 | 8.257812 | 2012-07-07 08:48:43.800 | 1.000000 | 3.0 | 1.341651e+09 | 0.08 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_5.21 | tm_5.21 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:00.600 | 0.390236 | 5.0 | 1.341652e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_5.22 | tm_5.22 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:10:55.640 | 0.152766 | 5.0 | 1.341652e+09 | 54.96 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_1.24 | tm_1.24 | 30.402637 | 40.763437 | 9.933594 | 2012-07-07 09:20:12.080 | 0.693933 | 1.0 | 1.341653e+09 | 556.44 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_5.24 | tm_5.24 | 30.353320 | 40.670625 | 1.960938 | 2012-07-07 09:27:10.120 | 0.332328 | 5.0 | 1.341653e+09 | 417.68 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_1.25 | tm_1.25 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 09:42:13.440 | 0.206876 | 1.0 | 1.341654e+09 | 903.28 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_2.27 | tm_2.27 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 09:46:04.920 | 0.341435 | 2.0 | 1.341654e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_5.26 | tm_5.26 | 30.397754 | 40.765937 | 10.644531 | 2012-07-07 09:59:27.240 | 0.186767 | 5.0 | 1.341655e+09 | 802.32 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_1.27 | tm_1.27 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 10:05:20.680 | 0.159305 | 1.0 | 1.341656e+09 | 353.36 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_4.0 | tm_4.0 | 30.322070 | 40.759375 | 2.164062 | 2012-07-07 10:16:39.800 | 1.000000 | 4.0 | 1.341656e+09 | 679.12 | True | 3.378762 | 1.691182 | -143.772340 | 2.733743 | NaN | NaN |
tm_5.27 | tm_5.27 | 30.427539 | 40.764375 | 9.273438 | 2012-07-07 10:41:34.400 | 0.335804 | 5.0 | 1.341658e+09 | 0.28 | True | 1.477016 | 1.028668 | 137.014524 | 1.263067 | NaN | NaN |
tm_0.30 | tm_0.30 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:15:32.640 | 0.298780 | 0.0 | 1.341660e+09 | 0.08 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_0.31 | tm_0.31 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 11:23:41.520 | 0.385650 | 0.0 | 1.341660e+09 | 0.04 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_1.30 | tm_1.30 | 30.331348 | 40.719062 | 0.996094 | 2012-07-07 12:13:39.320 | 0.266582 | 1.0 | 1.341663e+09 | 2997.80 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
tm_2.33 | tm_2.33 | 30.404590 | 40.762187 | 10.136719 | 2012-07-07 12:17:45.120 | 0.167869 | 2.0 | 1.341663e+09 | 245.44 | True | 1.204078 | 0.954942 | 138.520075 | 1.142778 | NaN | NaN |
tm_6.0 | tm_6.0 | 30.318896 | 40.676719 | 2.697266 | 2012-07-07 15:26:15.080 | 1.000000 | 6.0 | 1.341675e+09 | 11309.96 | True | 4.898208 | 2.005268 | 177.708818 | 3.423410 | NaN | NaN |
tm_3.14 | tm_3.14 | 30.445117 | 40.764375 | 8.054688 | 2012-07-07 16:50:34.840 | 0.255224 | 3.0 | 1.341680e+09 | 5059.76 | True | 2.982386 | 1.933982 | -169.556250 | 8.074210 | NaN | NaN |
tm_1.31 | tm_1.31 | 30.411914 | 40.793125 | 11.304688 | 2012-07-07 19:23:20.560 | 0.146581 | 1.0 | 1.341689e+09 | 9165.72 | True | 1.886675 | 1.435720 | -163.098388 | 1.737751 | NaN | NaN |
Compute earthquake magnitudes
This section is still work-in-progress! The goal is to use the moment magnitudes \(M_w\) that we computed in notebook 7 and the waveform peak amplitudes that we extracted during template matching in notebook 9 to compute local magnitudes for more earthquakes.
[27]:
for tp in template_group.templates:
if "Mw" in tp.aux_data:
self_detection = (final_catalog["tid"] == float(tp.tid)) & (final_catalog["cc"] > 0.999)
final_catalog.loc[self_detection, "Mw"] = tp.aux_data["Mw"]
final_catalog.loc[self_detection, "Mw_err"] = tp.aux_data["Mw_err"]
# final_catalog
[58]:
ref_events = final_catalog.dropna(subset=["Mw"])
ref_events
for event_id in events:
event = events[event_id]
event_id = f"tm_{event_id}"
tid = float(event.aux_data["tid"])
# print(tid)
subset = ref_events.tid == tid
if np.sum(subset) > 0:
peak_amp = event.aux_data["peak_amplitudes"]
Mls = []
for i, row in ref_events[subset].iterrows():
peak_amp_ref = events[row.name[3:]].aux_data["peak_amplitudes"]
ratios = (peak_amp/peak_amp_ref).flatten()
ratios = ratios[~np.isinf(ratios) & (ratios > 0.)]
Ml_diff = np.median(np.log10(ratios))
# Ml_i = row.Mw + 2./3. * Ml_diff
Ml_i = row.Mw + Ml_diff
Mls.append(Ml_i)
final_catalog.loc[event_id, "Ml"] = np.median(Mls)
final_catalog[["origin_time", "longitude", "latitude", "depth", "hmax_unc", "vmax_unc", "Mw", "Ml"]]
[58]:
origin_time | longitude | latitude | depth | hmax_unc | vmax_unc | Mw | Ml | |
---|---|---|---|---|---|---|---|---|
event_id | ||||||||
tm_1.0 | 2012-07-07 06:56:02.200 | 30.399707 | 40.764062 | 10.136719 | 1.886675 | 1.737751 | NaN | 2.586553 |
tm_3.1 | 2012-07-07 06:56:52.160 | 30.445117 | 40.764375 | 8.054688 | 2.982386 | 8.074210 | NaN | NaN |
tm_1.2 | 2012-07-07 07:07:45.440 | 30.404590 | 40.767187 | 10.238281 | 1.886675 | 1.737751 | NaN | 4.005050 |
tm_3.3 | 2012-07-07 07:09:59.680 | 30.445117 | 40.764375 | 8.054688 | 2.982386 | 8.074210 | NaN | NaN |
tm_0.3 | 2012-07-07 07:10:12.440 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_5.3 | 2012-07-07 07:10:20.000 | 30.397754 | 40.765937 | 10.644531 | 1.477016 | 1.263067 | NaN | 1.087575 |
tm_5.4 | 2012-07-07 07:10:39.160 | 30.398242 | 40.763125 | 10.289062 | 1.477016 | 1.263067 | NaN | 1.145118 |
tm_1.4 | 2012-07-07 07:10:53.640 | 30.413867 | 40.761875 | 10.898438 | 1.886675 | 1.737751 | NaN | 1.165784 |
tm_3.4 | 2012-07-07 07:11:07.280 | 30.413867 | 40.760625 | 10.898438 | 2.982386 | 8.074210 | NaN | NaN |
tm_5.6 | 2012-07-07 07:11:36.080 | 30.407520 | 40.762812 | 10.136719 | 1.477016 | 1.263067 | NaN | 1.124363 |
tm_0.8 | 2012-07-07 07:12:06.200 | 30.406543 | 40.762812 | 10.238281 | 1.204078 | 1.142778 | NaN | NaN |
tm_1.7 | 2012-07-07 07:14:25.000 | 30.400684 | 40.763437 | 10.136719 | 1.886675 | 1.737751 | 2.809426 | 2.809426 |
tm_1.8 | 2012-07-07 07:15:44.880 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 1.251298 |
tm_5.9 | 2012-07-07 07:18:17.040 | 30.397754 | 40.765937 | 10.644531 | 1.477016 | 1.263067 | NaN | 0.990920 |
tm_0.11 | 2012-07-07 07:22:16.240 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_0.12 | 2012-07-07 07:22:43.880 | 30.402148 | 40.744375 | 6.632812 | 1.204078 | 1.142778 | NaN | NaN |
tm_3.6 | 2012-07-07 07:23:08.320 | 30.393359 | 40.753750 | 2.671875 | 2.982386 | 8.074210 | NaN | NaN |
tm_1.9 | 2012-07-07 07:24:09.560 | 30.396777 | 40.769062 | 10.746094 | 1.886675 | 1.737751 | NaN | 1.027156 |
tm_5.11 | 2012-07-07 07:24:34.000 | 30.400684 | 40.765312 | 10.746094 | 1.477016 | 1.263067 | 2.604610 | 2.604610 |
tm_1.11 | 2012-07-07 07:26:09.920 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 1.171287 |
tm_1.12 | 2012-07-07 07:27:21.560 | 30.314746 | 40.715938 | 0.996094 | 1.886675 | 1.737751 | NaN | 0.869616 |
tm_5.13 | 2012-07-07 07:29:48.920 | 30.397754 | 40.765937 | 10.644531 | 1.477016 | 1.263067 | NaN | 1.004545 |
tm_3.8 | 2012-07-07 07:34:42.600 | 30.445605 | 40.765312 | 8.003906 | 2.982386 | 8.074210 | NaN | NaN |
tm_3.9 | 2012-07-07 07:35:58.000 | 30.445117 | 40.764375 | 8.054688 | 2.982386 | 8.074210 | NaN | NaN |
tm_3.10 | 2012-07-07 07:39:17.400 | 30.445117 | 40.764375 | 8.054688 | 2.982386 | 8.074210 | NaN | NaN |
tm_3.11 | 2012-07-07 08:00:23.360 | 30.445117 | 40.764375 | 8.054688 | 2.982386 | 8.074210 | NaN | NaN |
tm_1.14 | 2012-07-07 08:10:46.560 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 1.096352 |
tm_0.17 | 2012-07-07 08:15:48.880 | 30.338428 | 40.717969 | 1.021484 | 1.204078 | 1.142778 | NaN | NaN |
tm_0.18 | 2012-07-07 08:17:35.000 | 30.429492 | 40.763125 | 8.460938 | 1.204078 | 1.142778 | NaN | NaN |
tm_1.17 | 2012-07-07 08:30:27.240 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 1.085124 |
tm_2.19 | 2012-07-07 08:41:19.560 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_1.19 | 2012-07-07 08:45:27.600 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 1.000992 |
tm_2.21 | 2012-07-07 08:46:34.360 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_1.21 | 2012-07-07 08:47:00.560 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 1.066457 |
tm_3.12 | 2012-07-07 08:48:43.800 | 30.441211 | 40.764375 | 8.257812 | 2.982386 | 8.074210 | NaN | NaN |
tm_5.21 | 2012-07-07 09:10:00.600 | 30.397754 | 40.765937 | 10.644531 | 1.477016 | 1.263067 | NaN | 1.246354 |
tm_5.22 | 2012-07-07 09:10:55.640 | 30.397754 | 40.765937 | 10.644531 | 1.477016 | 1.263067 | NaN | 0.934968 |
tm_1.24 | 2012-07-07 09:20:12.080 | 30.402637 | 40.763437 | 9.933594 | 1.886675 | 1.737751 | NaN | 2.351699 |
tm_5.24 | 2012-07-07 09:27:10.120 | 30.353320 | 40.670625 | 1.960938 | 1.477016 | 1.263067 | NaN | 1.231549 |
tm_1.25 | 2012-07-07 09:42:13.440 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 0.972295 |
tm_2.27 | 2012-07-07 09:46:04.920 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_5.26 | 2012-07-07 09:59:27.240 | 30.397754 | 40.765937 | 10.644531 | 1.477016 | 1.263067 | NaN | 0.991324 |
tm_1.27 | 2012-07-07 10:05:20.680 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 0.886426 |
tm_4.0 | 2012-07-07 10:16:39.800 | 30.322070 | 40.759375 | 2.164062 | 3.378762 | 2.733743 | NaN | NaN |
tm_5.27 | 2012-07-07 10:41:34.400 | 30.427539 | 40.764375 | 9.273438 | 1.477016 | 1.263067 | NaN | 1.485605 |
tm_0.30 | 2012-07-07 11:15:32.640 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_0.31 | 2012-07-07 11:23:41.520 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_1.30 | 2012-07-07 12:13:39.320 | 30.331348 | 40.719062 | 0.996094 | 1.886675 | 1.737751 | NaN | 1.012254 |
tm_2.33 | 2012-07-07 12:17:45.120 | 30.404590 | 40.762187 | 10.136719 | 1.204078 | 1.142778 | NaN | NaN |
tm_6.0 | 2012-07-07 15:26:15.080 | 30.318896 | 40.676719 | 2.697266 | 4.898208 | 3.423410 | NaN | NaN |
tm_3.14 | 2012-07-07 16:50:34.840 | 30.445117 | 40.764375 | 8.054688 | 2.982386 | 8.074210 | NaN | NaN |
tm_1.31 | 2012-07-07 19:23:20.560 | 30.411914 | 40.793125 | 11.304688 | 1.886675 | 1.737751 | NaN | 0.854499 |